Introduction
We have another blog on making a headless RCP (Headless RCP - simple). In this article, we make the program into a standalone desktop RCP application.
Procedure
You need to make a “Product Configuration”.
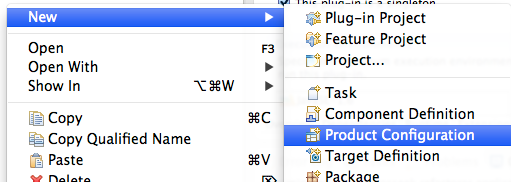
Select the application that we created before.
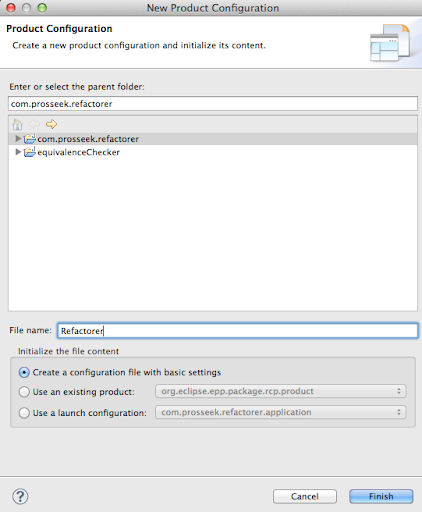
Setting the “Product Definition”.
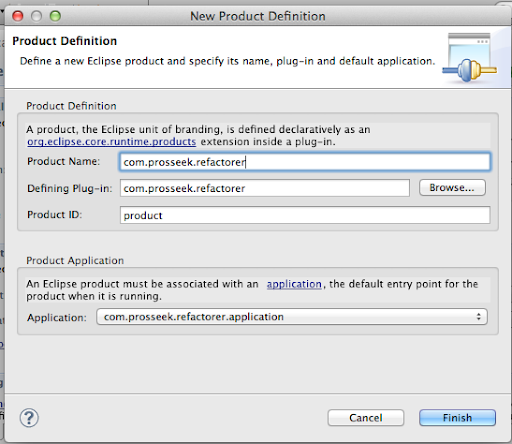
Don’t setup anything in ID.
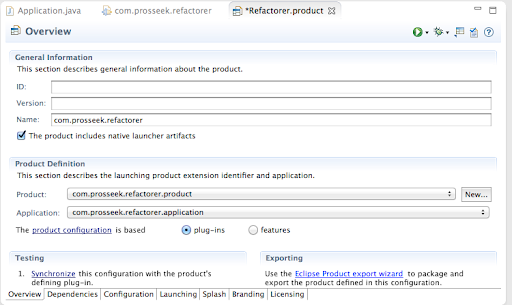
Update the MANIFEST.MF
Go to MANIFEST.MF, and you’ll see you already have an extension. Add property.
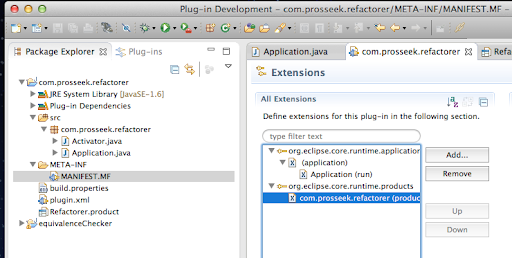
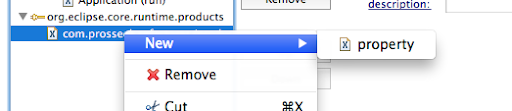
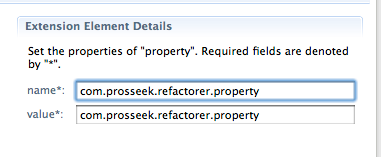
In Dependencies, you have to add the application you just created.
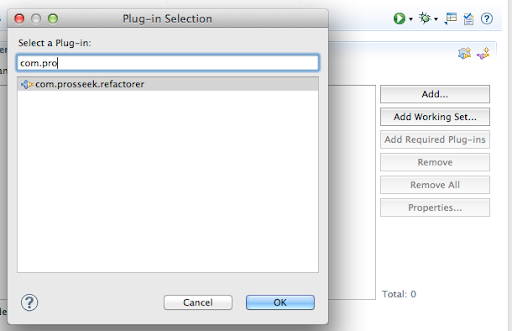
Then click “Add Required Plug-ins”.
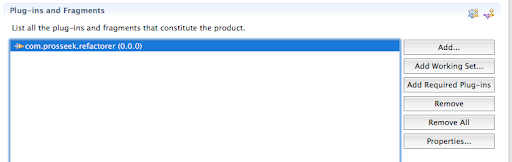
You’ll see all the required plugins are added.
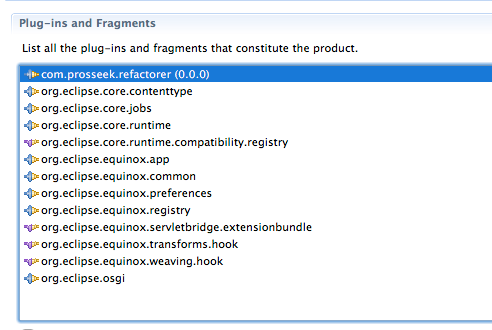
Click synchronize to check everything is OK.
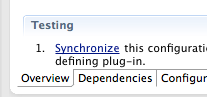
And then, you need to add all “.” and all the namespaces additional to your exported one in the “Runtime/Classpath”. Without this configuration you’ll have errors when you launch the standalone program even when your program works fine within the eclipse IDE.
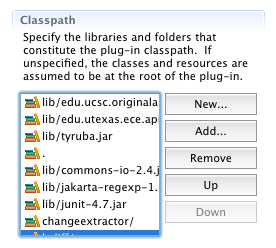
Exporting the headless RCP
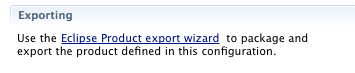
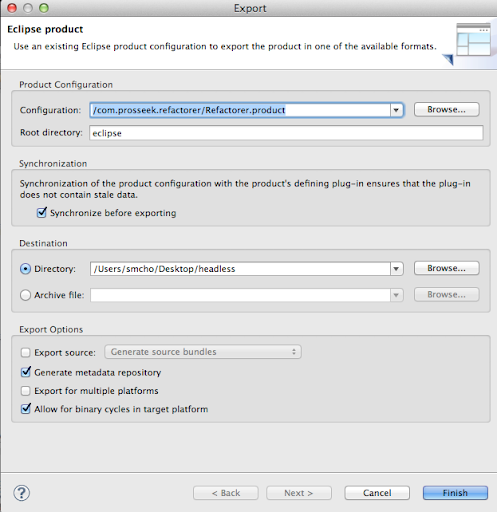
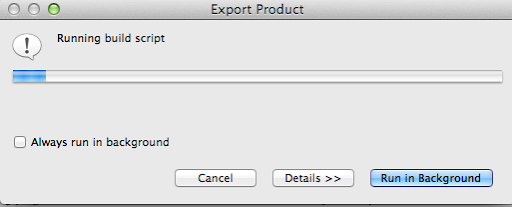
You’ll see that the standalone headless RCP is created in the directory you specified.
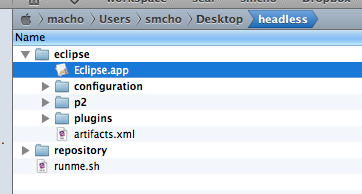
Executing standalone headless RCP
For execution, you can run “/DIRECTORY_TO_THE_RCP/Eclipse.app/Contents/MacOS/eclipse -data WORK_SPACE -clean”. You can make the shell script. Check that it also has the “-clean” parameter.
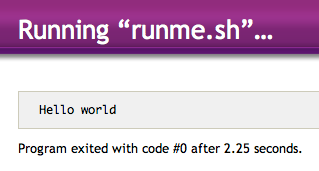
Run Configuration
You can create a run configuration so that you can launch the product within eclipse IDE.
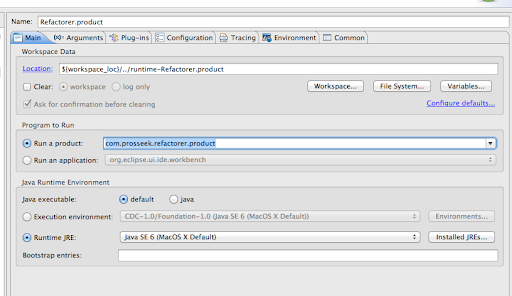
Add -clean parameter.
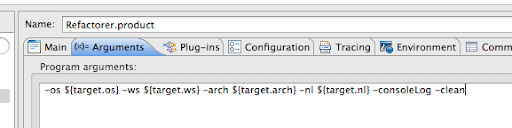
Add “Required Plug-ins”
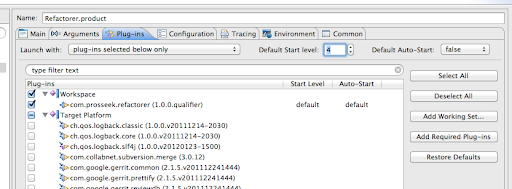
You can run to get the same result.
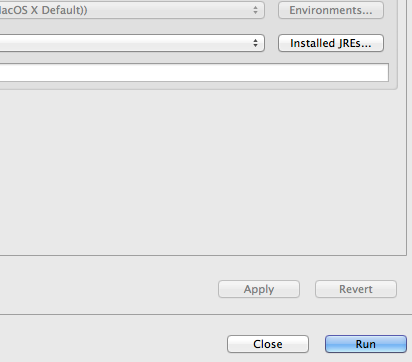
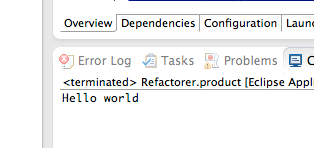
After the first launch, you have the product in the run button.
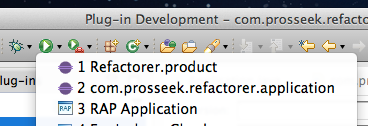
Issues and solutions
- It worked fine, but when you do something more and you suddenly have an error, the first thing you need to check is “Run Configurations”, then “Add Required Plug-ins”,
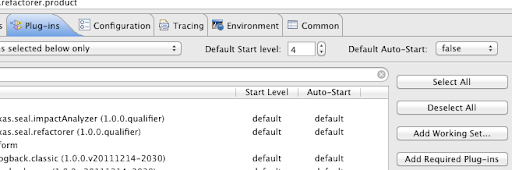
- Not found Activator
- You may have “org.osgi.framework.BundleException: The activator ABC.Activator for bundle ABC.refactorer is invalid” error when you launch the standalone binary. In that case, add “.” in the runtime class path.
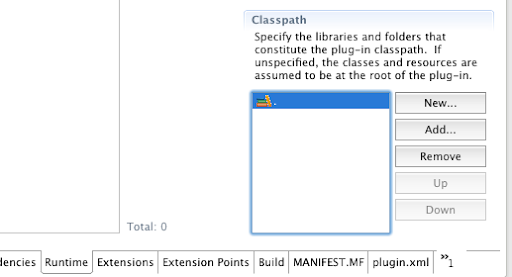
References