From wikipedia
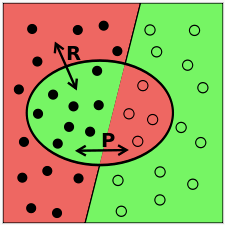
The left side is relevant domain and the circle means retrieved domain.
The perfect case can be the circle encloses only the left hand side, which is not always true, so we have precision and recall.

Precision means how precise we retrieved from samples. It focuses on not retrieving wrong samples. It’s the ratio between green circle and whole circle. Whole circle means what I retrieved and green circle means what I retrieved correctly.
So, precision says how accurate your retrieved result is. The more the less false positive.
When precision is low, it just means you include too many wrong samples.

Recall means how many samples you missed. It focuses on not missing correct samples. Think about the case car company recalls defected cars. It cares about the cars that it needs to recall, it doesn’t care about recalling non-defect cars. It’s the ratio between green circle and left side. Left area means the samples that I need to retrieve.
So, recall says did you good at not letting anything behind. The more the less false negative.
MacJournal article